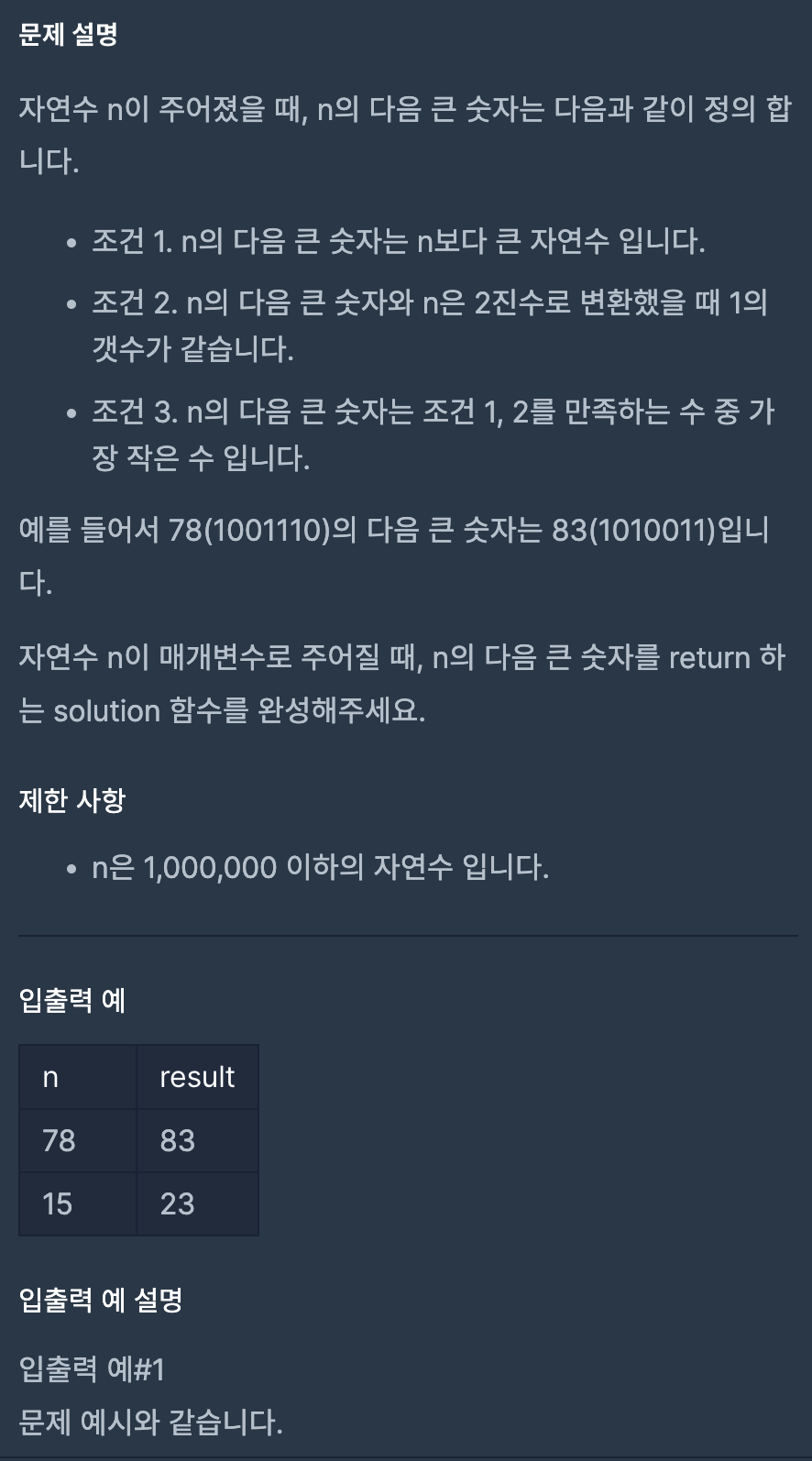

toBinaryString을 사용하면 int값을 이진수로 쉽게 변환할 수 있어 어렯지 않게 풀 수 있다.
하지만 bitCount라는 메소드를 쓰면 더 간결하게 표현할 수 있었다..
내가푼것
class Solution {
public int solution(int n) {
int answer = 0;
String binaryN = Integer.toBinaryString(n);
int count = 0;
for(int i = 0; i < binaryN.length(); i++) {
if(binaryN.charAt(i) == '1'){
count++;
}
}
while(true){
n++;
int count2 = 0;
String check = Integer.toBinaryString(n);
for(int i = 0; i < check.length(); i++){
if(count2 > count){
break;
}
if(check.charAt(i) == '1'){
count2++;
}
}
if(count == count2){
answer = n;
break;
}
}
return answer;
}
}
다른사람이 푼것
class TryHelloWorld
{
public int nextBigNumber(int n){
int a = Integer.bitCount(n);
while (Integer.bitCount(++n) != a) {}
return n;
}
public static void main(String[] args){
TryHelloWorld test = new TryHelloWorld();
int n = 78;
System.out.println(test.nextBigNumber(n));
}
}
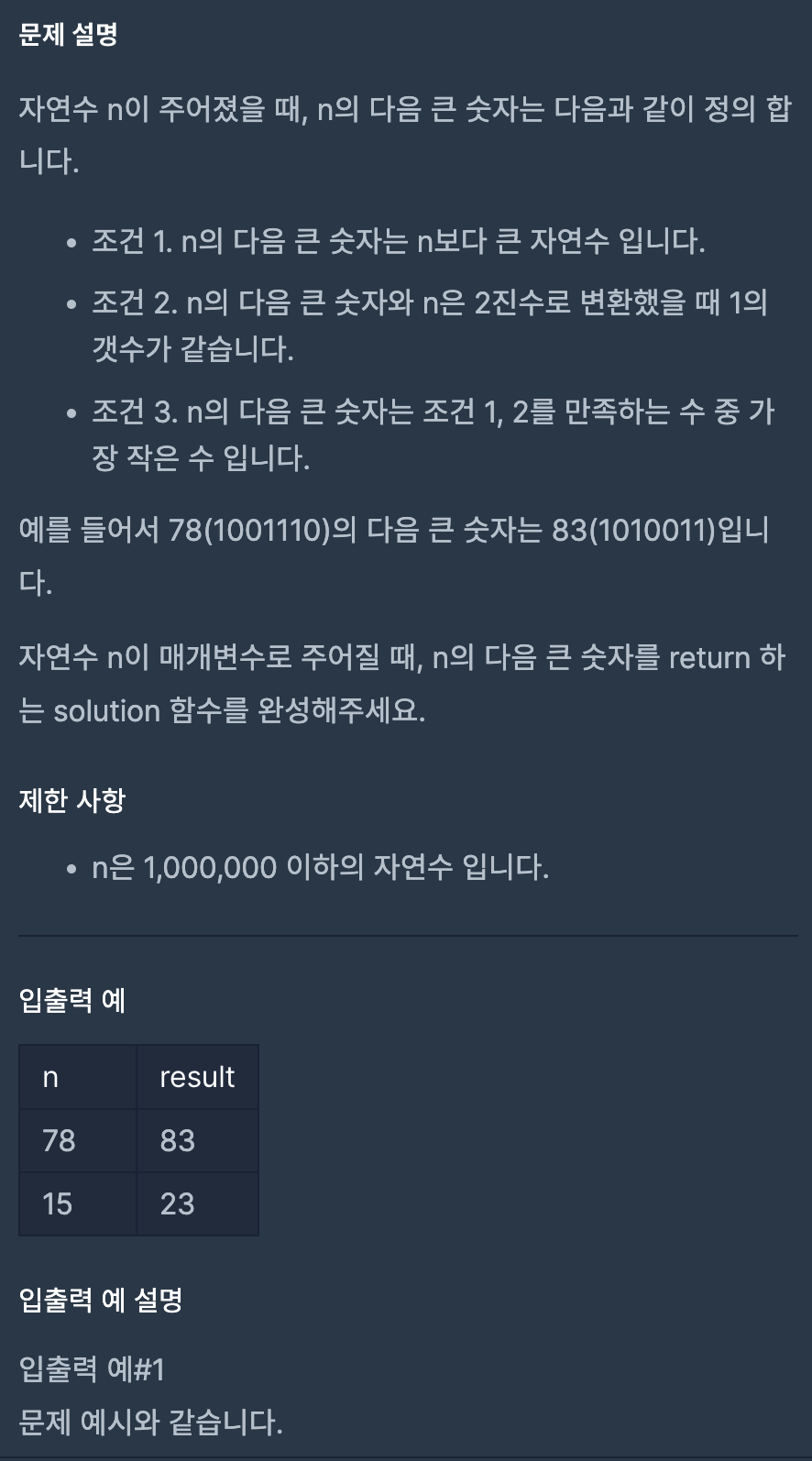

toBinaryString을 사용하면 int값을 이진수로 쉽게 변환할 수 있어 어렯지 않게 풀 수 있다.
하지만 bitCount라는 메소드를 쓰면 더 간결하게 표현할 수 있었다..
내가푼것
class Solution { public int solution(int n) { int answer = 0; String binaryN = Integer.toBinaryString(n); int count = 0; for(int i = 0; i < binaryN.length(); i++) { if(binaryN.charAt(i) == '1'){ count++; } } while(true){ n++; int count2 = 0; String check = Integer.toBinaryString(n); for(int i = 0; i < check.length(); i++){ if(count2 > count){ break; } if(check.charAt(i) == '1'){ count2++; } } if(count == count2){ answer = n; break; } } return answer; } }
다른사람이 푼것
class TryHelloWorld { public int nextBigNumber(int n){ int a = Integer.bitCount(n); while (Integer.bitCount(++n) != a) {} return n; } public static void main(String[] args){ TryHelloWorld test = new TryHelloWorld(); int n = 78; System.out.println(test.nextBigNumber(n)); } }